Now We're Nowhere In Her Thoughts, As She Dives Beneath The Waves
This is another one of my favourite quotes from "Girl and the Sea" by the Presets.
It represents everything I love in life... Moving on... Being the winner... Not thinking about people who have put you down, because, well, "now we're nowhere in her thoughts, as she dives beneath the waves".
Diving beneath the waves of course being the perfect metaphor for doing what you love...
Going to do a second consecutive Masters degree even though people told me I couldn't do it. Deciding to become a software engineer even though that wasn't the box that I fit into in so many people's minds. And reserving the right to talk about software engineering as much as I want to, and to be as passionate about it as I like, in spite of only having come across it at the age of 28,
because only I know how much I really love it, and how much it really means to me. ✨🎇🎇💖
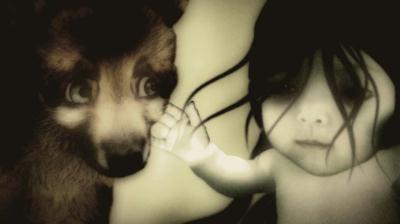 |
Some more beautiful art from the Girl and the Sea music video. |
3 Things About Arrays I Would Like To Come Back To
Just from yesterday, these are before I move on to learning the new content today.
1. Update Elements
So if you want to update an element in your array (an element in your array just means an item on your list), then it goes like this:
you need to put something like
nameOfArray[x] = 'newItem';
Where x is the position on your list - remember that it starts at 0!
so an example of this would be
let feverRaySongs = ['Triangle Walks', 'Coconut', 'Concrete Walls'];
feverRaySongs[1] = 'Seven';
console.log(feverRaySongs);
//Output: ['Triangle Walks', 'Seven', 'Concrete Walls']
So we have updated element 2 of the array.
Because arrays in JavaScript are zero-indexed.
2. Zero-Indexing
So just to confirm this
"the positions start counting from 0 rather than 1.
Therefore, the first item in an array will be 0".
3. Re-Assigning Elements
In JavaScript, variables that declared with let can be reassigned. Variables declared with const cannot. But with arrays, even if you declare the array with const, you can still update elements in the list as with the above example given. Let me give one more example of this, for it to really sink in for me.
const favouriteManchesterSpots = ['Ancoats', 'Fletcher Moss Park', 'Wythenshawe', 'Withington Golf Course'];
favouriteManchesterSpots[3] = 'The Northern Quarter';
console.log(favouriteManchesterSpots);
//Output: ['Ancoats', 'Fletcher Moss Park', 'Wythenshawe', 'The Northern Quarter'];
so as you can see you can do it even if the variable is assigned with const
Array Methods
.push()
The syntax for this is fairly simple.
array.push(item1, item2, ... itemN);
And it just adds it simply to the end of your list.
const fruits = ['strawberries', 'bananas']'
fruits.push('apples');
console.log(fruits);
//Output: ['strawberries, 'bananas', 'apples'];
Or one more example of multiple elements:
const trees = ['willow', 'ash', 'aspen', 'oak'];
trees.push('sequoia', 'birch', 'beech')
console.log(trees);
//Output: ['willow', 'ash', 'aspen', 'oak', 'sequoia', 'birch', 'beech']
.pop()
The .pop() removes and returns the last item of your array. So not only does it remove it but it also returns it and it can be assigned to a new value like so:
const trees = ['willow', 'ash', 'aspen', 'oak'];
const lonelyTree = trees.pop();
console.log(trees);
//Output: ['willow', 'ash', 'aspen']
console.log(lonelyTree);
//Output: oak
There are a lot of things you can do with an array - oh, omg, omg
.shift()
The .shift() method "removes and returns the first element of the array".
In that sense then it seems to be the same as the .pop() method but the opposite. I.e., it removes and then returns the first element instead of the last one.
However it is important to note that unlike the .pop() method, all of the elements on the list will shift down one place.
So normally the pattern in general of these methods is:
nameOfArry.method();
.unshift()
"Adds one or more elements to the beginning of an array and returns new lengths".
And so once again, it displaces all of the positions, of all of the elements.
.slice()
"The .slice() aray method returns a shallow copy of all or part of an array without modifying the original". Okay then, well what is a shallow copy? :(
Shallow Copies
Okay omg what wow this is FASCINATING!
"A shallow copy of an object is a copy whose properties share the same references (point to the same underlying values) as those of the source object from which the copy was made. As a result, when you change either the source or the copy, you may also cause the other object to change too — and so, you may end up unintentionally causing changes to the source or copy that you don't expect. That behavior contrasts with the behavior of a deep copy, in which the source and copy are completely independent."
Copied directly from the
MDN.
Back to the array methods
Got distracted there.
Back to the .slice() method (vol 2.0)
"The returned array contains the element specified by the first argument and all subsequent elements up to, but not including, the element specified by the second argument".
THE SECOND ARGUMENT DOES NOT GET INCLUDED.
A great example that Codecademy gave of this, which really makes it make sense, is this:
console.log(array.method(first, last+1));
Emphasis on the last+1 bit! It's AWESOME!!!
.slice() is non-mutating.
.indexOf()
Okay so right now I think the MDN explains it best. The MDN says:
"The indexOf() method returns the first index at which a given element can be found in the array, or -1 if it is not present".
This sounds a bit tricky but it's not. The 'index' basically just means what number on the list it is.
Remember that this:
const musicGenres = ['trance', 'electro', 'dance', 'hip-hop']
equates to the following:
0 - trance
1 - electro
2 - dance
3 - hip-hop
so if we searched for the letter 'l' then the expected output would be the number 1 because the letter 'l' first appears at index 1, or in electro.
Other array methods that seem like priorities but my brain needs a break from reading omg
This is based on the course and a Tech Lead who I asked at work today.
I can read these when my brain has space to read again. Right now I need to move on with my course.
ARRAYS THAT ARE MUTATED WITHIN FUNCTIONS RETAIN THESE MUTATIONS AFTER THESE FUNCTIONS ARE CALLED
Just putting that one out there to save myself a lot of time typing this out or explaining this somehow further/later down the line.
Nested Arrays
Arrays can contain other arrays.
"When an array contains another array it is known as a nested array".
Chaining Bracket Notation With Index Value
Okay that's it. The above sentence is the most technical thing that I have ever written in my life.
Examples of accessing nested elements using chaining bracket notation with index value.
(If I got a tattoo saying "chaining bracket notation with index value" would that be the nerdiest tattoo of all time? Yes? let's do it).
const favouriteThingsToEatTogether = [['pizza', 'pineapple'], ['nachos', 'sour cream']];
So I want to access 'sour cream', which is INDEX 1 (second item) of the SECOND ELEMENT WITHIN THE ARRAY OF MULTIPLE ARRAYS (so also INDEX 1).
So it's quite simple. I can access it like this.
console.log(favouriteThingsToEatTogether[1][1]);
//Output: sour cream
And that's it!!! It works!
Important and Interesting Things I Learned On Arrays Today
Here are two useful screenshots from my course. One:
And two:
And here are some exercises to practice with (maybe tomorrow):
One more important (but very important) point of reflection
So yesterday when I was talking to my boss I got 2 key messages:
1. I need to focus on mastering the basics of programming, the ones that are universal to all programming languages although I am learning them in JavaScript specifically.
2. I need to focus on improving my knowledge of operators.
Luckily, the MDN has me covered. I just need to figure out the best possible way of learning and approaching this thing.
I have raised this as a question on my company's internal questions channel for devs - let me see what they say and what they come back with.
Update:
I was told by Rosana to just focus on my course and continue practising operators within that.
Comments
Post a Comment